10-Days of learning - Day 3 - File Manipulation and System Information
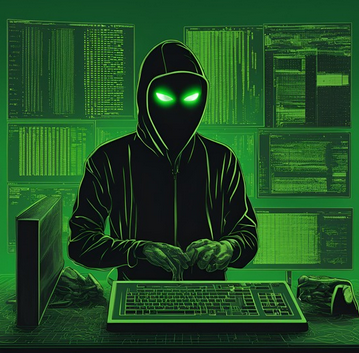
File Manipulation
File manipulation is an important aspect of malware development because it allows malware authors to perform various tasks such as stealing sensitive information, modifying system files, and persistence mechanisms.
1. Stealing Sensitive Information: Malware can be used to steal sensitive information from a system, such as passwords, financial data, or personal files. By reading and writing to files, malware can collect and exfiltrate this information.
2. Modifying System Files: Malware can modify system files to disrupt or change the behavior of the system. For example, a malware could modify system configuration files or registry keys to hijack system resources or disable security measures.
3. Persistence Mechanisms: Malware can use file manipulation to establish persistence on a system. This can be done by creating or modifying system files to ensure that the malware runs automatically when the system starts up or is restarted.
4. Evading Detection: Malware often needs to evade detection by modifying system files or registry keys to hide their presence on the system. This can be achieved by changing file attributes, timestamps, or file names.
5. Data Exfiltration: Malware can use file manipulation to exfiltrate data from a system. This can be done by writing sensitive information to files on the system or by copying files to external media or servers.
6. Malware Distribution: Malware can use file manipulation to distribute itself across a network. This can be done by copying malware files to network shares or by modifying system files to load malware from external sources.
File manipulation then, is a fundamental aspect of malware development because it allows malware authors to perform a variety of tasks that can compromise the security and integrity of a system. By understanding how file manipulation works and how malware can use it, developers can better protect against malware and create more robust security measures.
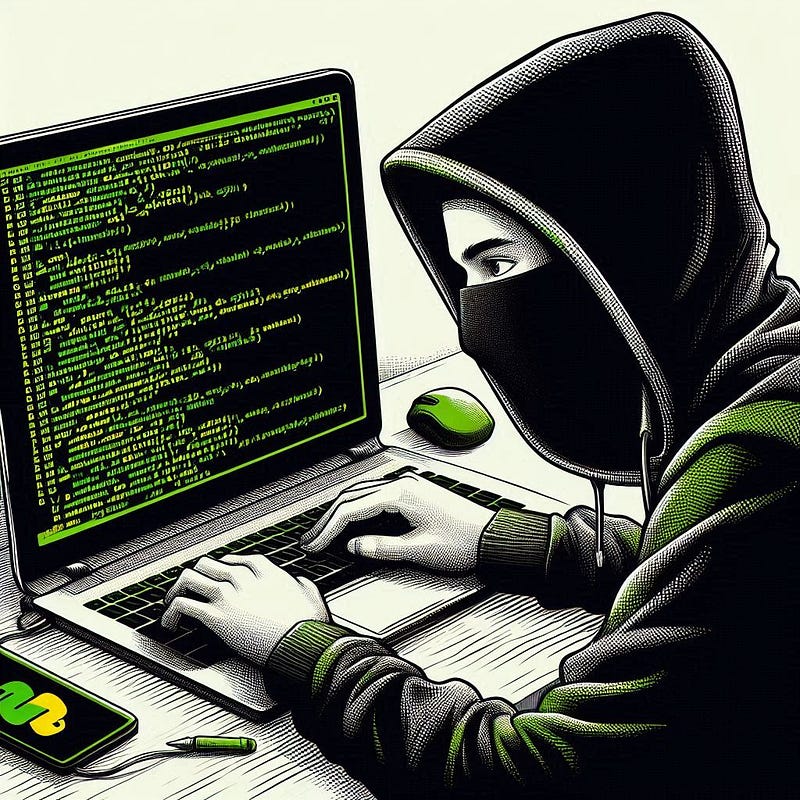
File Manipulation with Python
Python provides built-in functions for file manipulation. The open() function is used to open files, and the with statement ensures that the file is properly closed after use.
# Open a file in read mode
with open('malware.txt', 'r') as file:
content = file.read()
print(content)
# Open a file in write mode
with open('malware.txt', 'w') as file:
file.write("This is a malware file!")
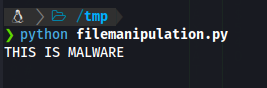
In the first example, we open a file in read mode, read its content, and print it. In the second example, we open a file in write mode and write some content to it.
File manipulation is not only enclosed on the read and modification but also in the creation, this process will be depending of the level of access the process has and the user who executes the malware, meaning, if a user with limited access wants to create a file within a high privileged folder this will not happen, that clarified lets dig into an example of file creation.
import os
# Function to create a malware file
def create_malware_file(filename):
with open(filename, 'w') as file:
file.write("This is a malware file!\n")
# Create a malware file
create_malware_file('malware.txt')
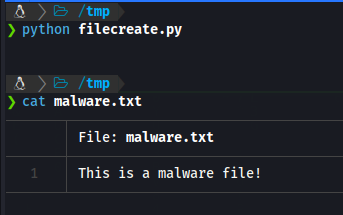
In this example, we define a function create_malware_file() that takes a filename as an argument. Inside the function, we use the open() function with the ‘w’ mode to create a file and write some content to it.
This function can be used in malware development to create files that can be used for various purposes such as stealing sensitive information, persistence, or data exfiltration.
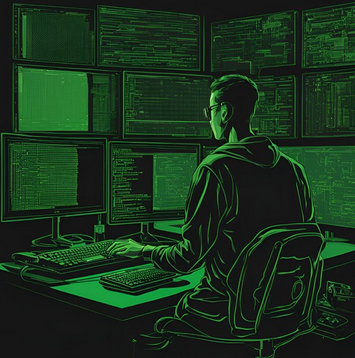
System Information Gathering
System information gathering is an important aspect of malware development because it allows malware authors to gather information about the system and its environment. Here are a few reasons why system information gathering is important for malware development:
1. Targeting Attacks: Malware can use system information to target specific systems or user groups. By gathering information about the system’s configuration, installed software, and user behavior, malware can identify vulnerable targets and focus their attacks accordingly.
2. Persistence Mechanisms: Malware can use system information to establish persistence on a system. This can be done by creating or modifying system files or registry keys to ensure that the malware runs automatically when the system starts up or is restarted.
3. Anti-Analysis Techniques: Malware can use system information to evade detection by modifying system files, registry keys, or environment variables. This can include techniques like packing, obfuscation, and anti-debugging measures.
4. Stealth and Covert Communication: Malware can use system information to establish covert communication channels or to blend in with normal system activity. This can be done by modifying network traffic, file names, or timestamps.
5. Stealing Sensitive Information: As mentioned before, malware can use system information to steal sensitive information from the system, such as passwords, financial data, or personal files. By analyzing system logs and configuration files, malware can identify sensitive data and exfiltrate it.
System information gathering then, is a fundamental aspect of malware development because it allows malware authors to gather information about the system and its environment, which can be used to identify targets, evade detection, and steal sensitive information. By understanding how system information can be gathered and how malware can use it, developers can better protect against malware and create more robust security measures.
Using Python OS library
import os
# Get current working directory
current_directory = os.getcwd()
print("Current directory:", current_directory)
# Get system environment variables
env_variables = os.environ
print("Environment variables:", env_variables)
# Get system version
system_version = os.uname()
print("System version:", system_version)
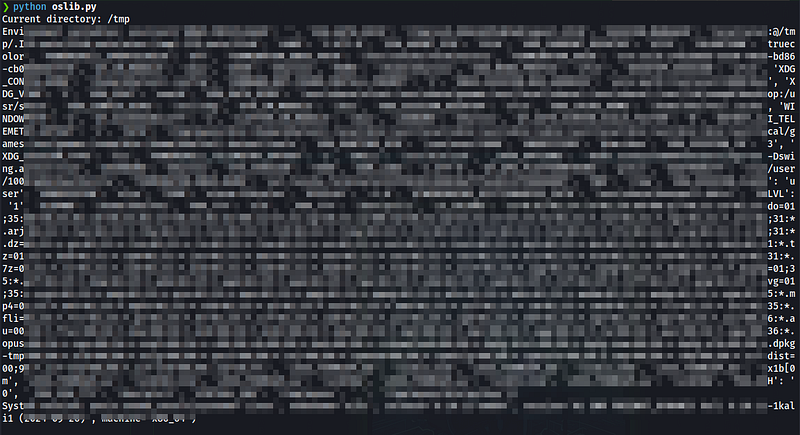
The function os.getcwd() retrieves the current working directory where the Python script is being executed. This can be useful for understanding the file system context or performing file operations relative to the script’s location. The result is printed to the console.
The os.environ object provides a dictionary of the system’s environment variables. These variables can contain important system configurations, paths, or other sensitive information like API keys or user credentials. The function prints all the environment variables, which can be used to gather information about the system’s configuration.
The function os.uname() returns information about the system, such as the operating system name, node name, release, version, and machine architecture. This information can be useful for identifying the system’s platform, which may be needed for compatibility checks or when performing system-specific operations.
PSutil
import psutil
# Get CPU information
cpu_info = psutil.cpu_times()
print("CPU Times:", cpu_info)
# Get memory information
memory_info = psutil.virtual_memory()
print("Memory Info:", memory_info)
# Get disk usage information
disk_usage = psutil.disk_usage('/')
print("Disk Usage:", disk_usage)
# Get network information
network_info = psutil.net_io_counters()
print("Network Info:", network_info)

In this example, we use the psutil library to gather various system information. We use the cpu_times() function to get CPU usage information, virtual_memory() to get memory information, disk_usage() to get disk usage information, and net_io_counters() to get network information.
The psutil library provides a wide range of functions for monitoring system resources, process management, and network usage. It can be used in various ways to gather information about the system and its environment, which can be used by malware authors to identify targets, evade detection, and steal sensitive information, for more information about the library consult the official documentation at https://pypi.org/project/psutil/.
Remember to practice ethical hacking and follow the law when performing malware analysis and development. Any misuse of the content is strictly prohibited.
I hope this article provides you with a comprehensive understanding of file manipulation and system information in Python for malware development.
