10-Days of learning - Day 2 - Basic Python Scripting for Malware
Python is a high-level programming language that is widely used for various tasks, including web development, data analysis, artificial intelligence, and security. In this article, we will explore the basics of Python scripting for malware development.
Introduction
Malware is a type of software designed to damage or disrupt a computer system. Python can be used for various purposes, including malware development. It is a high-level language that is easy to learn and read, making it a popular choice for malware authors.
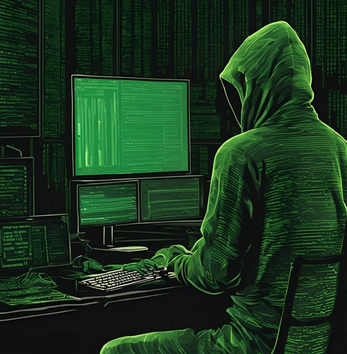
Basic Python Scripting for Malware
Python scripting allows malware authors to automate tasks and perform system-level operations. Here are some basic examples of Python scripting for malware:
If ,else and elif statement
Python supports various control flow statements, including if, else, elif, and nested if statements. The if statement is used to execute a block of code only if a certain condition is true. The else statement is used to execute a block of code when the condition in the if statement is false. The elif statement is used to check multiple conditions and execute a block of code when one of the conditions is true. Here’s an example:
# Example of an if-elif-else statement
age = 15
if age >= 21:
print("You are an adult.")
elif age >= 18:
print("You are a young adult.")
else:
print("You are a minor.")
In this example, if the age is greater than or equal to 21, “You are an adult.” is printed. If the age is greater than or equal to 18 but less than 21, “You are a young adult.” is printed. Otherwise, “You are a minor.” is printed.

do Loop
Python doesn’t have a built-in do…while loop like some other languages, but you can simulate it using a while loop. The key feature of a do…while loop is that the code block will execute at least once before checking the condition.
count = 0
while True:
print("Count:", count)
count += 1
if count >= 5:
break # Exiting the loop after 5 iterations
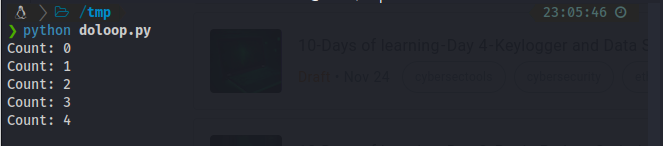
while Loop
A while loop repeatedly executes a block of code as long as a specified condition is True.
count = 0
while count < 5:
print("Count:", count)
count += 1
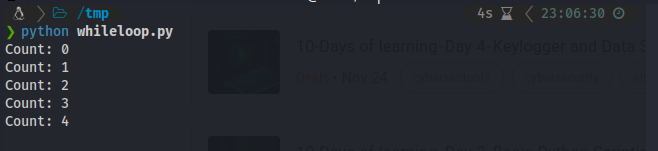
In this example, we start by initializing a variable count
with the value 0
. We then enter a while loop that will continue executing as long as the condition count < 5
is true. Inside the loop, we print the current value of count
, increment the value of count
by 1
using the +=
operator, and then check the condition again.
The loop will continue executing until count
reaches 5
, at which point the condition count < 5
becomes False
, and the loop terminates.
try, except, and finally
try Block: This is where you write the code that might raise an exception. If an error occurs, the flow of control moves to the except block.
except Block: This block allows you to handle specific exceptions. If the code in the try block raises an error that matches the exception specified, the code inside the except block will execute.
finally Block: This block contains code that will run no matter what — whether an exception was raised or not. It’s typically used for cleanup actions, such as closing files or releasing resources.
def divide_numbers(num1, num2):
try:
result = num1 / num2 # Attempt to divide
print("Result:", result)
except ZeroDivisionError: # Handle division by zero
print("Error: Cannot divide by zero!")
finally:
print("Execution complete.") # This will always run
# Example usage
divide_numbers(10, 2) # This will succeed
divide_numbers(10, 0) # This will raise an exception
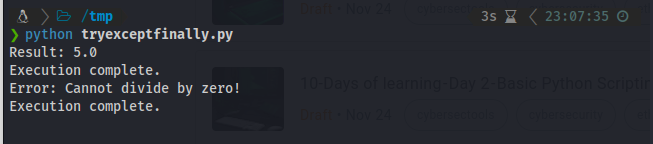
System Information Gathering
Python provides modules like os and sys to gather system information.
import os
import sys
# Get current working directory
current_directory = os.getcwd()
print("Current directory:", current_directory)
# Get system environment variables
env_variables = os.environ
print("Environment variables:", env_variables)
# Get system version
system_version = sys.version
print("System version:", system_version)
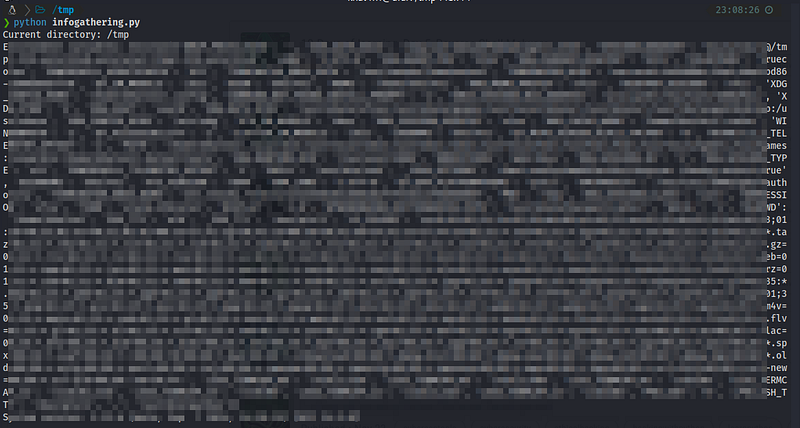
Requests
Python’s requests library is a powerful tool for making HTTP requests. It allows you to send HTTP requests to web servers and retrieve responses. Here’s a simple example of how to use the requests library for making HTTP requests:
import requests
# Make a GET request to a URL
response = requests.get('https://example.com')
# Check if the request was successful
if response.status_code == 200:
print("Request successful")
print("Response content:")
print(response.text)
else:
print("Request failed")
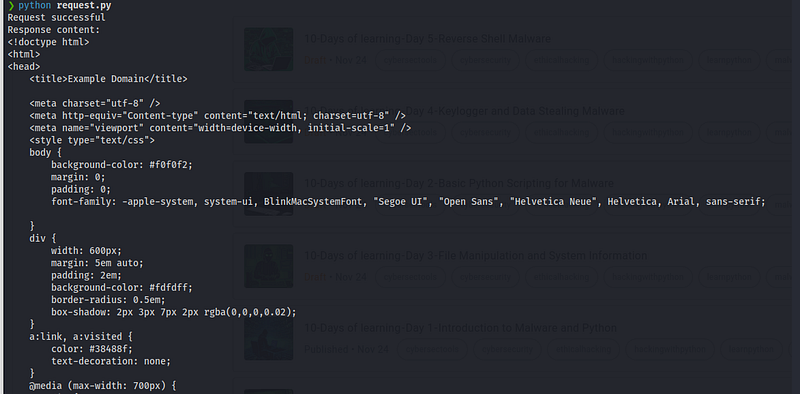
In this example, we use the requests.get() method to make a GET request to the specified URL. We then check the status code of the response. If the status code is 200 (indicating a successful request), we print the response content. If the status code is anything else, we print “Request failed.”
This example demonstrates the basic usage of the requests library for making HTTP requests. You can extend this example to include more complex scenarios, such as handling authentication, sending data, or parsing JSON responses.
Subprocess
Python’s subprocess module is used to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. It allows you to run external programs and scripts from within a Python script.
import subprocess
# Define the command to run
command = ['nmap', '-sV', '192.168.1.0/24']
# Run the command and get the output
output = subprocess.run(command, capture_output=True, text=True)
# Print the output
print(output.stdout)
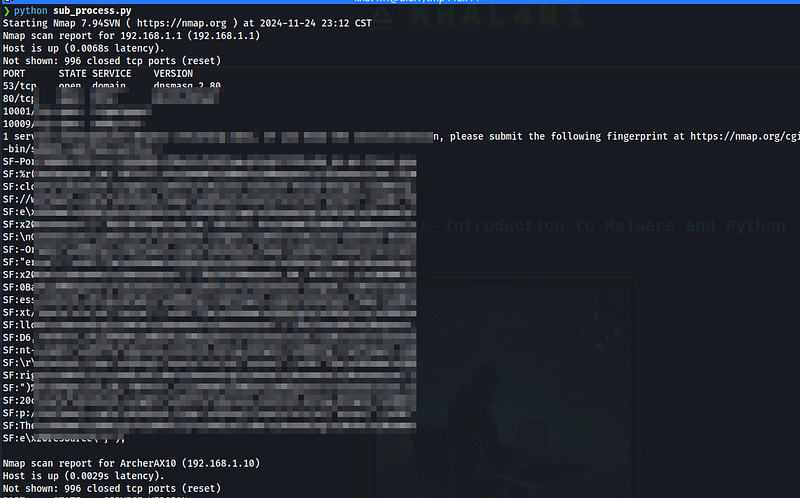
In this example, we use the subprocess.run() method to execute the nmap command with the -sV option (version detection) and the target network range 192.168.1.0/24. The capture_output=True option ensures that the output is captured, and the text=True option decodes the output to a string.
The print(output.stdout) line prints the output of the nmap command, which lists the open ports and their corresponding services on the specified network range.
The subprocess module can be used to run any external program or script from within a Python script. It provides a high-level interface for creating and managing processes.
PSutil
Python’s psutil library is a powerful tool for system monitoring, process management, and resource usage analysis. It provides a wide range of functions for retrieving information about the system, processes, and network usage.
import psutil
# Get network interfaces
net_if_addrs = psutil.net_if_addrs()
# Debugging: print the interfaces to check if any are found
if net_if_addrs:
print("Network Interfaces Found:")
for interface_name, interface_addresses in net_if_addrs.items():
print(f"Interface: {interface_name}")
for address in interface_addresses:
print(f" Address: {address.address} (Family: {address.family})")
else:
print("No network interfaces found.")
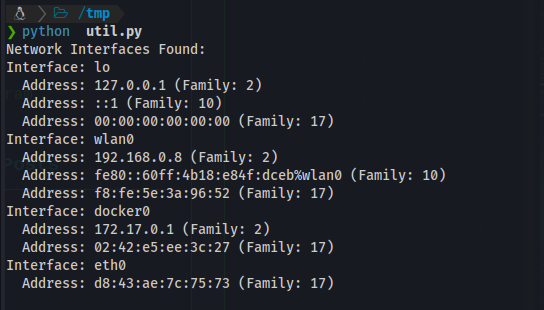
In this example, we use the psutil.net_if_addrs()
function to get a dictionary of network interfaces and their addresses. This function returns a dictionary where the keys are the interface names (e.g., eth0
, wlan0
), and the values are lists of address objects associated with each interface.
We then iterate over each network interface in the dictionary. For each interface, we print the interface name and, for each address associated with that interface, we print the IP address, address family (e.g., AF_INET
for IPv4), netmask, and broadcast IP.
This allows us to see detailed information about the network interfaces available on the system.
The psutil
library can be used in various ways to monitor system resources, manage processes, and perform advanced network-related operations. In this case, it provides a simple and effective way to gather network interface details programmatically.
Conclusion
Python scripting is a powerful tool for creating malware because it allows you to automate tasks and perform system-level operations. Malware authors can use Python to create scripts that can steal sensitive information, install backdoors, or gain unauthorized access to a system.
Remember to practice ethical hacking and follow the law when performing malware analysis and development. Any misuse of the content is strictly prohibited.
I hope this article provides you with a comprehensive understanding of basic Python scripting for malware development. Let me know if you have any further questions!
